Re: Idea for virtual memory allocator: yea or nay? [verdict:
Posted: Sun Jan 31, 2021 8:27 am
An O(n) algorithm would be like this
An O(1) algorithm would be like this
The difference, an O(1) algorithm always executes in the same amount of time no matter what. It is also much faster in must circumstances, as a block of code will execute once only. An O(n) algorithm is a bitmap, for example, you loop through every bit in the map to find a free one. A free list is a O(1) algorithm, it has its info right there. No looping needed.
Graphed, you can see the difference. Here are some links to what these algorithm types look like graphed
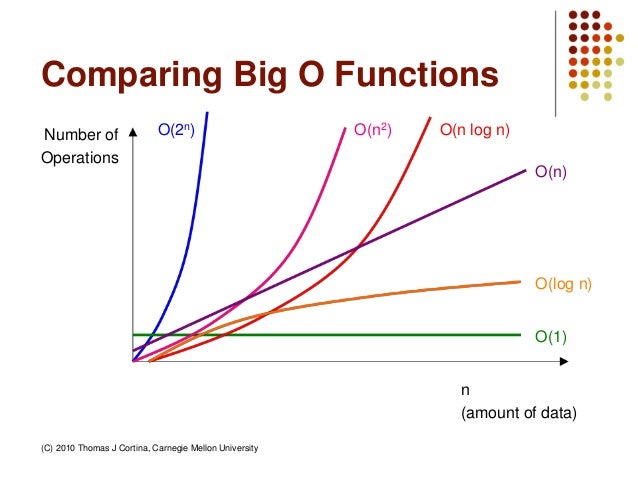
Code: Select all
for(int i = 0; i < num; ++i)
{
do something;
}
Code: Select all
do something;
Graphed, you can see the difference. Here are some links to what these algorithm types look like graphed
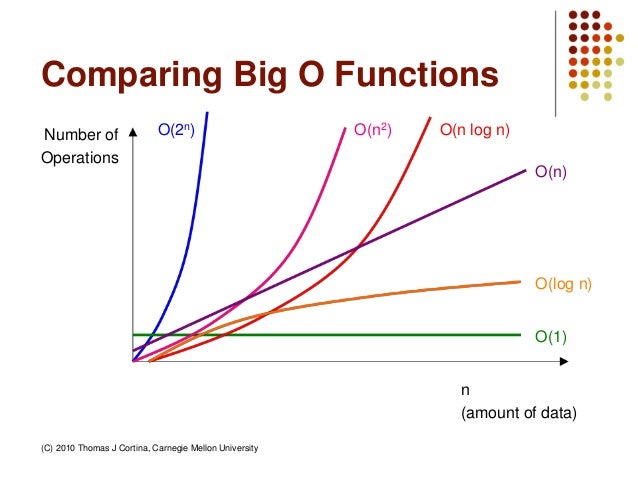