Code: Select all
void _sysprintf(char* str, uint8_t bgcolor, uint8_t forecolor) {
static uint16_t* VideoMemory = (uint16_t*)0xb8000;
static uint8_t x = 0, y = 0;
uint8_t text_color = ((bgcolor) << 4) | (forecolor & 0x0F);
for(int i = 0; str[i] != '\0'; ++i)
{
switch(str[i])
{
case '\n':
y++;
x = 0;
break;
case '\b':
if (x <= 0)
{
x = 0;
}
else {
x -= 1;
}
VideoMemory[80*y+x] = (VideoMemory[80*y+x] & 0xFF00) | ' ';
break;
default:
VideoMemory[80*y+x] = (VideoMemory[80*y+x] & (text_color << 8)) | str[i];
x++;
break;
}
if(x >= 80) {
y++;
x = 0;
}
if (y >= 25) {
for(y = 0; y < 25; y++)
for(x = 0; x < 80; x++)
VideoMemory[80*y+x] = (VideoMemory[80*y+x] & 0xFF00) | ' ';
x = 0;
y = 0;
}
}
cursor_update(x, y);
}
Code: Select all
_sysprintf("A", 0x00, 0x00);
_sysprintf("B", 0x00, 0x01);
_sysprintf("C", 0x00, 0x02);
_sysprintf("D", 0x00, 0x03);
_sysprintf("E", 0x00, 0x04);
_sysprintf("F", 0x00, 0x05);
_sysprintf("G", 0x00, 0x06);
_sysprintf("H", 0x00, 0x07);
_sysprintf("I", 0x00, 0x08);
_sysprintf("J", 0x00, 0x09);
_sysprintf("K", 0x00, 0x10);
_sysprintf("L", 0x00, 0x11);
_sysprintf("M", 0x00, 0x12);
_sysprintf("N", 0x00, 0x13);
_sysprintf("O", 0x00, 0x14);
_sysprintf("!", 0x00, 0x15);
printf("\n");
_sysprintf("!", 0x00, 0x00);
_sysprintf("A", 0x01, 0x00);
_sysprintf("B", 0x02, 0x00);
_sysprintf("C", 0x03, 0x00);
_sysprintf("D", 0x04, 0x00);
_sysprintf("E", 0x05, 0x00);
_sysprintf("F", 0x06, 0x00);
_sysprintf("G", 0x07, 0x00);
_sysprintf("H", 0x08, 0x00);
_sysprintf("I", 0x09, 0x00);
_sysprintf("J", 0x10, 0x00);
_sysprintf("K", 0x11, 0x00);
_sysprintf("L", 0x12, 0x00);
_sysprintf("M", 0x13, 0x00);
_sysprintf("N", 0x14, 0x00);
_sysprintf("O", 0x15, 0x00);
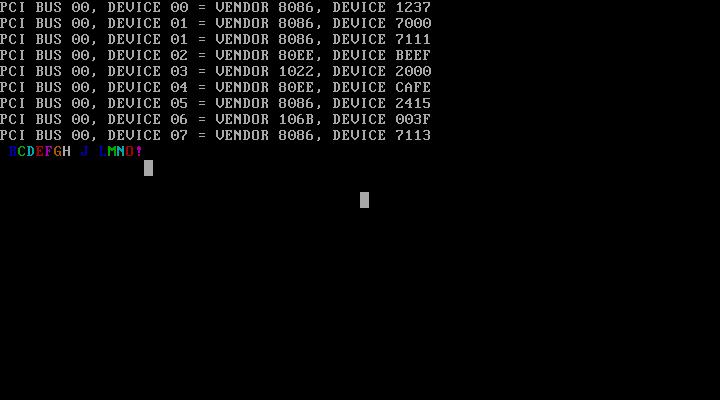
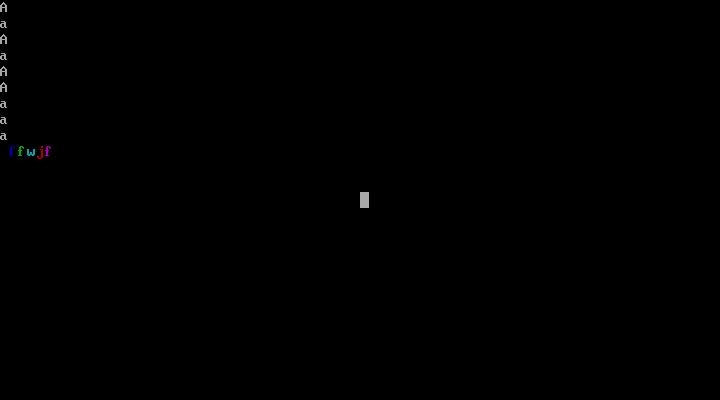
I tried many different solutions, I was looking up for answers and nothing worked. I think this must be some kind of very silly mistake, am I shifting the bytes in wrong way?