i have no problems working with floppy disks. here's a screen shot of it trying to enumerate partitions on a hard drive. it's a single FAT16 partition, but it's reading everything all wrong and apparently it's because it's not calculating the correct starting point of the volume on the disk.
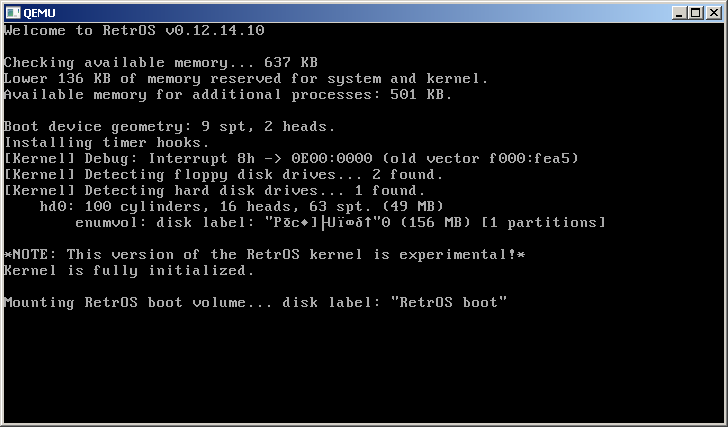
and i had it print out some debug info to vtty 2, and it's like it's calculating the CHS incorrectly? it should be starting to read the partition at offset 32256, but it apparently thinks it starts at 24064. i've verified that viewing the drive image in a hex editor.
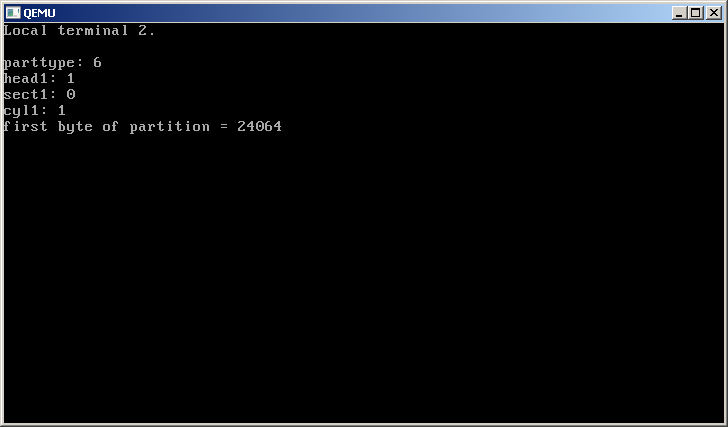
here's the relevant code. go easy on me, i'm relatively new to C. i'm a BASIC veteran. still learning the C ropes. the disk reading routines which aren't pasted here should be just fine, they're flawless on floppies. you can see in the first screenshot the last line gets the disk label string correctly.
Code: Select all
void readparts(uint8 disknum) {
struct structpart {
uint8 status;
uint8 head1;
uint8 sectcyl11;
uint8 sectcyl12;
uint8 parttype;
uint8 head2;
uint8 sectcyl21;
uint8 sectcyl22;
uint32 lbastart;
uint32 sectorcount;
} tmppart;
uint8 mbr[512];
uint32 templong;
uint16 tempword, tempvol;
uint8 tempbyte = 0;
sprintf(tmpstr, " enumvol: "); ttyprint(maintty, tmpstr);
if (readdisk(disknum, 0, 512, &mbr[0])) {
sprintf(tmpstr, "error reading disk!\r\n"); ttyprint(maintty, tmpstr);
return;
} else if (mbr[510]!=0x55 || mbr[511]!=0xAA) {
sprintf(tmpstr, "invalid partition table!\r\n"); ttyprint(maintty, tmpstr);
return;
}
for (tempvol=0; tempvol<4; tempvol++) {
if (readdisk(disknum, 0x1BE+(tempvol*16), 16, &tmppart)==0)
if ((tmppart.status==0 || tmppart.status==0x80) && (tmppart.parttype==0x04 || tmppart.parttype==0x06)) {
volmap[lastvol].cyl1 = (((uint16)tmppart.sectcyl12&0xC0)<<2)|(uint16)tmppart.sectcyl11;
volmap[lastvol].head1 = tmppart.head1;
volmap[lastvol].sect1 = tmppart.sectcyl11&63;
volmap[lastvol].cyl2 = (((uint16)tmppart.sectcyl22&0xC0)<<2)|(uint16)tmppart.sectcyl21;
volmap[lastvol].head2 = tmppart.head2;
volmap[lastvol].sect2 = tmppart.sectcyl21&63;
volmap[lastvol].exist = 1;
volmap[lastvol].sects = tmppart.sectorcount;
volmap[lastvol].drive = disknum;
//the "heads+1" in the next 2 lines are correct, the variable stores that and the cylinder count as the max addressable value, not the absolute total number.
volmap[lastvol].startsect = ((uint32)volmap[lastvol].cyl1*((uint32)disk[disknum].heads+1)*(uint32)disk[disknum].spt)+(((uint32)volmap[lastvol].head1)*(uint32)disk[disknum].spt)+(uint32)volmap[lastvol].sect1-1;
volmap[lastvol].endsect = ((uint32)volmap[lastvol].cyl2*((uint32)disk[disknum].heads+1)*(uint32)disk[disknum].spt)+(((uint32)volmap[lastvol].head2)*(uint32)disk[disknum].spt)+(uint32)volmap[lastvol].sect2-1;
tmppart.sectorcount = volmap[lastvol].endsect - volmap[lastvol].startsect;
sprintf(tmpstr, "\r\nparttype: %x\r\nhead1: %u\r\nsect1: %u\r\ncyl1: %u\r\n", tmppart.parttype, volmap[lastvol].head1, volmap[lastvol].sect1, volmap[lastvol].cyl1);
ttyprint(maintty+1, tmpstr);
initvol(lastvol++); //this calls a rotine in the FAT driver module that examines the volume boot record, works great on floppies.
sprintf(tmpstr, "%u (%u MB), ", tempvol, (tmppart.sectorcount*512)/1048576);
ttyprint(maintty, tmpstr);
tempbyte++;
}
}
sprintf(tmpstr, "%c%c [%u partitions]\r\n", 8, 8, tempbyte); ttyprint(maintty, tmpstr);
}
Code: Select all
struct structvolmap {
uint8 drive;
uint8 drivepartnum;
uint32 startsect;
uint32 endsect;
uint16 spt;
uint8 heads;
uint8 sects;
uint16 cyl1;
uint8 sect1;
uint8 head1;
uint16 cyl2;
uint8 head2;
uint8 sect2;
char exist;
} volmap[0x100];