here is a screenshot
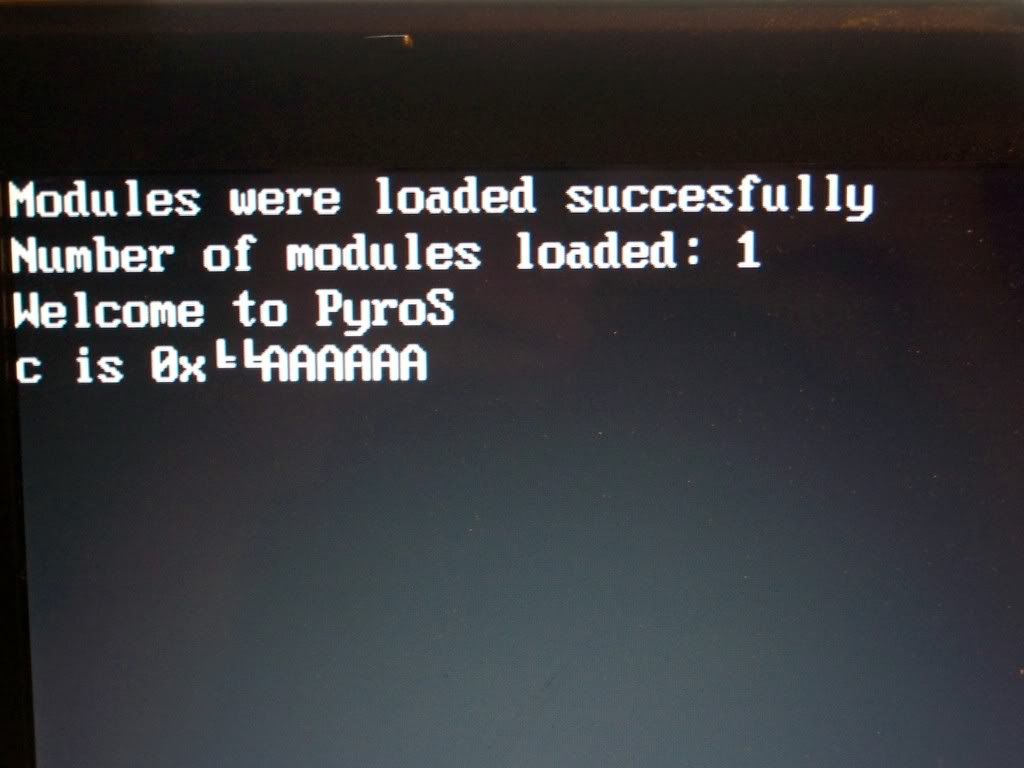
and the relevant code
printf
Code: Select all
void Printf(char *fmt,...)
{
va_list args;
char number[10];
int num;
char buf;
int buffer;
int buffer2;
va_start(args,fmt);
while(*fmt!=NULL)
{
if(*fmt=='%')
{
fmt++;
switch(*fmt)
{
case 'i':
case 'd':
case 'u':
itoa(va_arg(args,int),number);
Put(number);
break;
case 'c':
putchar((unsigned char)va_arg(args,int));
break;
case 'x':
buffer=va_arg(args,int);
buffer2=buffer;
buffer=buffer<<28;
buffer=buffer>>28;
putchar(return_hex(buffer));
buffer=buffer2;
buffer=buffer<<24;
buffer=buffer>>28;
putchar(return_hex(buffer));
buffer=buffer2;
buffer=buffer<<20;
buffer=buffer>>24;
putchar(return_hex(buffer));
buffer=buffer2;
buffer=buffer<<16;
buffer=buffer>>20;
putchar(return_hex(buffer));
buffer=buffer2;
buffer=buffer<<12;
buffer=buffer>>16;
putchar(return_hex(buffer));
buffer=buffer2;
buffer=buffer<<8;
buffer=buffer>>12;
putchar(return_hex(buffer));
buffer=buffer2;
buffer=buffer<<4;
buffer=buffer>>8;
putchar(return_hex(buffer));
buffer=buffer2;
buffer=buffer|0x0F;
putchar(return_hex(buffer));
break;
case 's':
Put(va_arg(args,char*));
break;
default:
putchar(*fmt);
break;
}
fmt++;
}
else
{
putchar(*fmt);
fmt++;
}
}
va_end(args);
}
Code: Select all
char return_hex(unsigned short num)
{
switch(num)
{
case 0x00:
return '0';
break;
case 0x01:
return '1';
break;
case 0x02:
return '2';
break;
case 0x03:
return '3';
break;
case 0x04:
return '4';
break;
case 0x05:
return '5';
break;
case 0x06:
return '6';
break;
case 0x07:
return '7';
break;
case 0x08:
return '8';
break;
case 0x09:
return '9';
break;
case 0x0A:
return 'A';
break;
case 0x0B:
return 'B';
break;
case 0x0C:
return 'C';
break;
case 0x0D:
return 'D';
break;
case 0x0E:
return 'E';
break;
case 0x0F:
return 'F';
break;
default:
break;
}
}
Code: Select all
void _main(struct MULTIBOOT *mbd,unsigned int magic)
{
char *code;
int c=0xAF;
clrscr(BLACK,BLACK);
set_color(WHITE,BLACK);
set_echo(1);
if((mbd->flags&0x08)==0x08)
{
Printf("Modules were loaded succesfully\n");
Printf("Number of modules loaded: %d\n",mbd->mods_count);
code=(char*)mbd->mods_addr;
}
else
{
Printf("Modules were not succesfully loaded\n");
}
idt_install();
gdt_install();
Printf("Welcome to PyroS\n");
Printf("c is 0x%x",c);
for(;;);
}